State Management Showdown: React vs. Vue 3 – Global & Local Strategies Unleashed
@technology
3 months ago
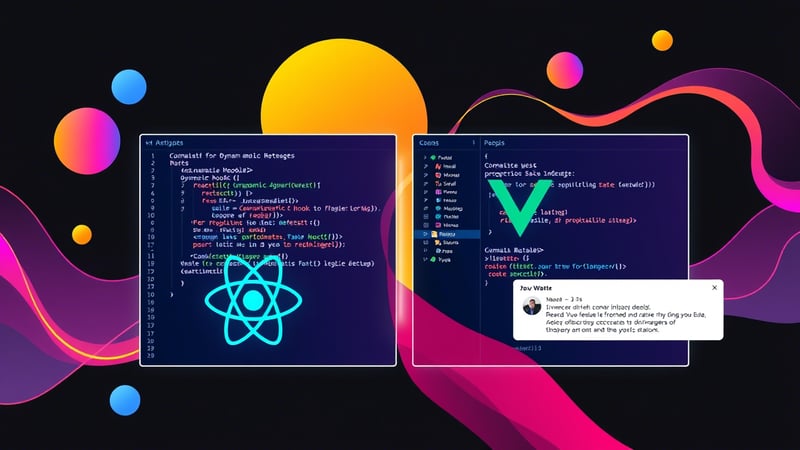
State Management Showdown: React vs. Vue 3 – Global & Local Strategies Unleashed
State management is like the secret sauce to building robust, scalable, and maintainable applications. In this blog, we're diving deep into the dynamic world of state management, comparing how React and Vue 3 do it. From React's flexible set of tools to Vue 3's innovative features like Pinia for global state and props + emit for local state, we’ll explore the pros, cons, and playful nuances of each framework.
Introduction
Imagine you're at a cookout, and your dish is missing that magic ingredient—state management! Both React and Vue 3 bring their own flavor to the table:
React: Offers a variety of state management approaches (local state, Context API, Redux, MobX, etc.). It’s flexible and powerful but sometimes requires a bit of extra plumbing.
Vue 3: Simplifies the process with built-in patterns, using props + emit for local state communication and Pinia as a lightweight, straightforward global state management tool.
In this blog, we'll compare state management by putting React and Vue 3 head-to-head, with energetic examples and hands-on code snippets to illustrate each approach in action.
Global State Management
React's Global State Options
React doesn’t enforce a single state management solution, so you’ve got a whole toolkit at your disposal. Let’s consider two popular methods:
Context API: Suitable for light-weight global state management, great for avoiding prop-drilling.
Redux: A more robust and mature solution for larger applications that require a centralized state store and powerful debugging features.
Example: Global State with React Context API
// GlobalStateContext.js
import React, { createContext, useState, useContext } from 'react';
const GlobalStateContext = createContext();
export const GlobalStateProvider = ({ children }) => {
const [user, setUser] = useState({ name: 'John Doe', age: 30 });
return (
<GlobalStateContext.Provider value={{ user, setUser }}>
{children}
</GlobalStateContext.Provider>
);
};
export const useGlobalState = () => useContext(GlobalStateContext);
// App.jsx
import React from 'react';
import { GlobalStateProvider, useGlobalState } from './GlobalStateContext';
const UserProfile = () => {
const { user } = useGlobalState();
return <div>{`User: ${user.name} (${user.age} years old)`}</div>;
};
const App = () => (
<GlobalStateProvider>
<h1>React Global State with Context API</h1>
<UserProfile />
</GlobalStateProvider>
);
export default App;
Vue 3's Global State using Pinia
Vue 3 leverages the modern Pinia library as its official state management solution. Pinia is lightweight, modular, and integrates flawlessly with Vue's reactivity system.
Example: Global State with Pinia in Vue 3
// stores/userStore.js
import { defineStore } from 'pinia';
export const useUserStore = defineStore('user', {
state: () => ({
user: { name: 'Jane Doe', age: 28 }
}),
actions: {
updateUser(newUser) {
this.user = newUser;
}
},
});
<!-- components/UserProfile.vue -->
<template>
<div>
{{ user.user.name }} ({{ user.user.age }} years old)
</div>
</template>
<script setup>
import { useUserStore } from '../stores/userStore.js';
const user = useUserStore();
</script>
Pinia’s API is straightforward with defineStore making it incredibly simple to create separate modules for your global state.
Local State Management
While global state is essential for sharing data broadly across components, local state plays a key role in component-specific interactions.
React’s Local State with useState
React’s useState
hook is a staple when it comes to handling local state within a component.
Example: Local State in React
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Current Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
Vue 3’s Local State with Props and Emit
Vue takes a slightly different approach. For local state, data flows from parent to child through props, and events can be sent back up using emit. This unidirectional data flow makes it super easy to reason about the state within your application.
Example: Local State in Vue 3 (Props + Emit)
<!-- ParentComponent.vue -->
<template>
<div>
<h1>Local State with Vue's Props and Emit</h1>
<Counter :initialCount="5" @count-changed="handleCountChanged" />
</div>
</template>
<script setup>
import Counter from './Counter.vue';
const handleCountChanged = (newCount) => {
console.log('The count was updated to:', newCount);
};
</script>
<!-- Counter.vue -->
<template>
<div>
<p>Local Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script setup>
import { ref, watch, defineProps, defineEmits } from 'vue';
const props = defineProps({
initialCount: {
type: Number,
default: 0
}
});
const emit = defineEmits(['count-changed']);
const count = ref(props.initialCount);
const increment = () => {
count.value++;
emit('count-changed', count.value);
};
// If you want to watch changes in the prop itself (for example, if initialCount might change)
watch(() => props.initialCount, (newVal) => {
count.value = newVal;
});
</script>
The beauty of Vue’s approach lies in its simplicity:
Props pass data down to child components.
Emit sends events back up to notify the parent of changes.
Comparison Highlights
Ease of Integration:
React: Offers multiple global state options, but requires choosing and possibly integrating external libraries.
Vue 3: With Pinia as the official global state solution and a natural props + emit pattern for local state, Vue streamlines the setup.
Boilerplate and Learning Curve:
React: The Context API is relatively simple, but more robust solutions like Redux can introduce extra boilerplate.
Vue 3: Pinia’s API and built-in local state communication keep things minimal and intuitive.
Reactivity and Performance:
React: Leveraging hooks like
useState
anduseReducer
offers fine-grained reactivity, though sometimes re-render optimization may require additional effort.Vue 3: Vue’s reactivity system, combined with Pinia’s out-of-the-box performance optimizations, often results in smoother state changes without much additional planning.
Final Thoughts
Both React and Vue 3 offer robust state management strategies, but your choice might depend on your project’s needs and your team's familiarity with each framework. React provides flexibility and a plethora of options, while Vue 3, with its built-in patterns like props + emit and Pinia for global state, makes achieving predictable state management delightfully straightforward.
In the end, whether you're a seasoned React wizard or a Vue enthusiast, mastering state management in your framework of choice is a game-changer. Happy coding, and may your states always be in perfect sync! 🚀✨
You may also like:
D o you want to connect with people who share your interests, passions, and goals? Do you want to express yourself, showcase your talents, and discover new opportunities? Do you want to enjoy a safe and enjoyable platform that offers everything you need for your daily computing needs? If you answered yes to any of these questions, then you should join NXplan.com, the new all-in-one social platform that features blog, messaging, chat, inbox, and marketplace.
NXplan.com is more than just a social network.
It’s a social ecosystem that allows you to create, communicate, and collaborate with others in a variety of ways. You can:Create your own blog and share your thoughts, opinions, and experiences with the world. You can also follow other bloggers and get inspired by their content. Message your friends and family and stay in touch with them. You can also make new friends and join groups that match your interests.
Chat with other users and have fun conversations. You can also join live events and webinars and learn from experts and influencers. Manage your inbox and organize your emails. You can also send and receive files, photos, and videos with ease. Explore the marketplace and find products and services that suit your needs. You can also sell your own products and services and earn money.
NXplan.com is designed to provide you with a safe and enjoyable platform that respects your privacy and security.
You can: Control your own data and decide who can see and access your information. Report and block any abusive or inappropriate content or users. Enjoy a spam-free and ad-free environment that does not track or sell your data. Access the platform from any device and any browser, without any downloads or installations. NXplan.com is free to join and use, and you can get started in minutes. All you need is a valid email address and a password. You can also customize your profile and settings to make it your own.Ready to give it a try?
Join NXplan.com today and discover a new way of socializing online. You’ll be amazed by what you can do and who you can meet on NXplan.com. Don’t miss this opportunity to join the next big thing in social media. Register now and start your NXplan journey.Technology is nothing. What's important is that you have a faith in people, that they're basically good and smart, and if you give them tools, they'll do wonderful things with them
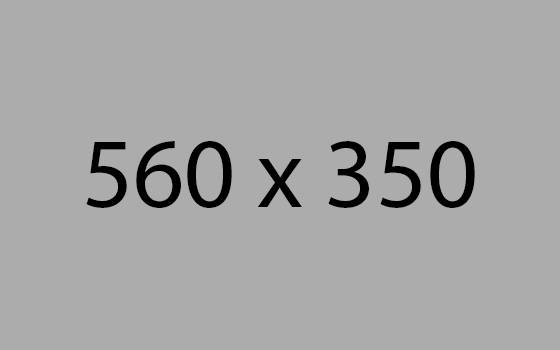
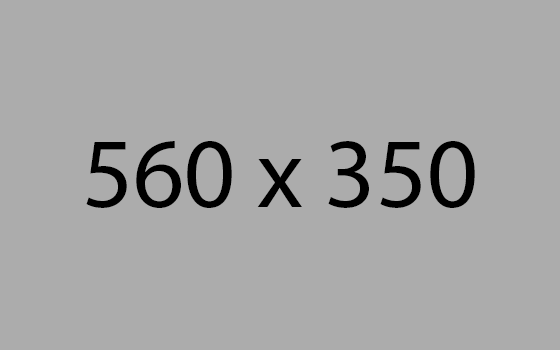